Table of Contents
Toggle
Introduction
If you are gearing up for a Python interview, congratulations on your first step towards an exciting journey in the world of software development. Python is a versatile and powerful programming language and as you know, is immensely popular for its simplicity, readability, and vast ecosystem of libraries and frameworks. As a beginner, facing an interview might feel overwhelming. We have compiled a list of 32 essential Python interview questions. These questions will provide valuable insights into core Python concepts and test your knowledge about its applications. So, let’s dive in!
Related Post: Top 14 Trending Python Applications in 2024

Top Python Interview Questions for Beginners
1. What is Python?
Python is a high-level, interpreted programming language. It is also known as a general-purpose programming language with a vast collection of tools and libraries that can be used to develop applications for many domains.
2. What are some features of Python language?
Python is a free, open source programming language with a simple, easy-to-read syntax. Applications built with Python are easy to maintain. Moreover, the programming language supports third-party packages, which makes it possible to modify and reuse the code.
3. What type of language is Python?
Python is a high-level, interpreted, programming language. It has a straightforward syntax that is easy to read and write. Python supports procedural, object-oriented, and functional programming. It is known as a beginner-friendly language and is suitable for a wide range of applications.
4. What is a dynamically typed language?
In programming languages, typing means type-checking, which is verifying and enforcing the data types of variables in a program during compile-time or runtime. Python is dynamically typed, which means that the type of a variable is interpreted at runtime and can change during the execution of a program.
5. Why is Python an interpreted language?
Python is executed line by line at runtime. In other words, it runs directly from the source code and does not include machine-level code before runtime. The source code written by the programmer is converted into an intermediate language, which is again translated into machine language.
6. What is the difference between lists and tuples in Python?
In Python, sequence data types that can store a collection of objects are called Lists and Tuples. The objects stored in lists and tuples can have different data types. Lists are represented with square brackets [‘sara’, 6, 0.19], while tuples are represented with parantheses (‘ansh‘, 5, 0.97). Lists are mutable. They can be modified, appended or sliced on the go. On the other hand, tuples are immutable and cannot be modified in any way.
7. What is pickling and unpickling?
These are serializing and deserializing processes in Python. Pickling is a serializing process used to convert a Python object into a byte stream. The function used for pickling is pickle.dump().
Unpickling is the reverse of pickling. It is the process of reconstructing the original object from that byte stream. The function used for unpickling is pickle.load().
8. What is PEP 8?
Python Enhancement Proposal of PEP 8 is an official design document that documents important details about Python. It can be referred to for information about the community and style guidelines for Python Code. Also, programmers should adhere to the PEP 8 guidelines when making ‘contributions to the Python open-source community.
9. What are Python modules?
In Python, a module is a file containing Python code. It may include definitions of functions, classes, and variables, or runnable code. Modules are a way to organize and structure Python code by breaking it into smaller, more manageable pieces.
10. What is the difference between modules and libraries?
A module is a single file containing Python code. A library is a collection of pre-written code and resources, often consisting of multiple modules. A module can be an individual file, but a library is typically a more extensive collection of related functionalities organized in a modular fashion.
11. What are decorators?
Decorators are functions that let you add functionality to an existing function in Python without changing the structure of the function itself. It is a very useful functionality because decorators are flexible and used for tasks like logging, memoization, access control, and more. Decorators use the @decorator syntax before a function or method definition.
12. What is type conversion in Python?
When you convert one data type into another, it is called type conversion.
- tuple() – This function is used to convert to a tuple.
- list() – This function is used to convert any data type to a list type.
- set() – This function returns the type after converting to set.
- int() – This converts any data type into an integer type.
- float() – This converts any data type into float type
- dict() – This function is used to convert a tuple of order (key, value) into a dictionary.
- str() – This is used to convert an integer into a string.
- ord() – This converts characters into integer
- hex() – This converts integers to hexadecimal
- oct() – This converts integer to octal
- complex(real,imag) – This function converts real numbers to complex(real,imag) number.
13. What is Scope in Python?
A scope is a block of code where an object in Python remains relevant. The concept of scope is related to the concept of namespace. A Python scope determines where in your program a name is visible. The dictionaries that map names to objects are namespaces.
14. What are Python namespaces?
Namespaces in Python are names assigned to objects. With each created object, its name, along with space, gets created. Namespace in Python is maintained like a dictionary where the key is the namespace, and the value is the address of the object. There are four types of namespaces in Python:
- Built-in namespace: These namespaces have all the built-in objects in Python and are available whenever Python is running.
- Global namespace: These are namespaces for all the objects created at the level of the main program.
- Enclosing namespaces: These namespaces are at the higher level or outer function.
- Local namespaces: These namespaces are at the local or inner function.
15. What is __init__ in Python?
In Python, __init__ is a special method, often referred to as the “initializer” or “constructor.” It is automatically called when an instance of a class is created. The primary purpose of __init__ is to initialize the attributes of the object. The __init__ is similar to constructors in object-oriented programming (OOP) terminology.
16. What is PYTHON PATH?
It is an environment variable within the Python programming language that allows users to define supplementary directories for Python to search modules and packages. It helps Python locate the necessary files to import during code execution. PYTHON PATH enhances code reusability and makes importing modules easier because of its ability to organize and structure Python projects.
17. What are local variables and global variables in Python?
Global Variables are variables declared outside a function or in global space. These variables can be accessed by any function in the program. Local Variables refer to any variable declared inside a function. This variable is present in the local space and not in the global space.
18. What are the tools that help to find bugs or perform static analysis?
PyChecker and Pylint can be used as static analysis and linting tools. PyChecker raises alerts for code issues and their complexity and can also help in finding bugs in Python source code files. Pylint can check for the module’s coding standards and support different plugins to enable custom features to meet this requirement.
19. What are the mutable built-in types in Python?
Mutable built-in types are:
- List
- Sets
- Dictionaries
- Immutable built-in types
- Strings
- Tuples
- Numbers
20. What are the immutable built-in types in Python?
Immutable built-in types are:
- Strings
- Tuples
- Numbers
21. What is Lambda in Python?
Lambda is an anonymous function in Python that can accept any number of arguments but can only have a single expression. It is generally used in situations that require an anonymous function for a short time period.
22. What is the difference between arrays and lists?
Arrays in Python only contain elements of the same data types. Lists in Python contain elements of different data types. The data type of array should be homogeneous. It is a thin wrapper around C language arrays and consumes far less memory than lists. The data type of lists can be heterogeneous. It has the disadvantage of consuming large memory.
23. Describe the split(), sub(), and subn() methods found within Python’s ‘re’ module.
Python’s “re” module offers three methods to modify strings.
- Split() uses a regex pattern to “split” a given string into a list.
- Sub() finds all substrings where the regex pattern matches and then replaces them with a different string
- Subn() is like sub() and returns the new string along with the no. of replacements.
24. What do negative indexes represent, and what is their purpose?
Negative indexes are the indexes from the end of the list or tuple or string. They are used to access elements from the end of a sequence, such as a list or a string. Negative indexes are a convenient way to access elements in reverse order without explicitly calculating the index based on the length of the sequence.
25. What is a multiple line comment in Python?
A multiple line comment in Python is a comment that can be seen in more than one line. All the lines to be commented on should be prefixed by a #. There is also a shortcut to comment multiple lines. Hold the ctrl key and left click in every place wherever you want to include a # character and type a # just once. This will comment all the lines where you introduced your cursor.
26. How can you shuffle the elements of a list in Python?
In Python, you can shuffle the elements of a list using the shuffle function from the random. Here are ways that can be used to shuffle a list:
- Use sorted()
- Use random.shuffle()
- Use random.sample()
- Use the Random Selection Method
- Use Fisher-Yates shuffle Algorithm
- Use itertools.permutations() function
- Use NumPy
27. How does break, continue, and pass work?
The break statement terminates the loop immediately, and the control flows to the statement after the body of the loop.
The continue statement terminates the current iteration of the statement. Then it skips the rest of the code in the current iteration, and the control flows to the next iteration of the loop.
The pass keyword in Python fills up empty blocks and is like an empty statement represented by a semicolon in languages such as Java, C++, Javascript, etc.
28. Explain the requirement of indentation in Python.
Indentation specifies a block of code. All code within loops, classes, and functions is specified within an indented block. It is usually done using four space characters. If your code is not indented necessarily, it will not execute accurately and will throw errors as well.
29. Explain Python packages. Why do we need them?
Python packages help with modular programming. With packages, it is possible to have hierarchal structuring of the module namespace using dot notation. Packages help avoid clashes between module names. It is easy to create a package since it makes use of the system’s inherent file structure.
30. Mention any two differences between loc and iloc
Loc indexing is label-based, whereas iloc indexing is integer position-based. With respect to slicing, loc end label is inclusive in the range, whereas for iloc, it is exclusive in the range.
31. Why do we need NumPy in Python?
NumPy is a Python library that helps with efficient numerical computing. It has high-performance multidimensional array objects and tools for working with these arrays. NumPy is memory-efficient and provides extensive mathematical functionality. Its arrays are the basis of most Python-based data science applications.
32. How to install Python on Windows and set a path variable?
- Install Python from this link: https://www.Python.org/downloads/
- After this, install it on your PC. Look for the location where PYTHON has been installed on your PC using the following command on your command prompt: cmd Python.
- Then go to advanced system settings, add a new variable, and name it PYTHON_NAME and paste the copied path.
- Look for the path variable, select its value and select ‘edit’.
- Add a semicolon towards the end of the value if it’s not present, and then type %PYTHON_HOME%
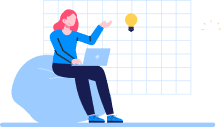
Don't miss out on your chance to work with the best!
Apply for top job opportunities today!

Conclusion
As you reach the end of this blog, you have taken significant steps in preparing for your Python interview. Do remember that these questions are meant to assess your current knowledge and inspire continuous learning. Python is a dynamic programming language, and with the ever-evolving tech landscape, being a continuous learner will benefit you the most. Whether you are aiming for your first Python-related role or advancing in your career, reflecting on these questions will help you a great deal. Best of luck with your Python interviews!
FAQs
Here are a few top Python-related careers you can look at:
- Python Developer
- Data Analyst
- Systems Engineer
- Data Scientist
- Machine Learning Engineer
Here are 5 companies that have used Python:
- Netflix
- Amazon
- Dropbox
You will benefit a lot from learning R and Python if you are a Data Scientist. Data science is all about crunching numbers in different ways, and Python has a vast collection of tools, frameworks, and libraries for various applications in Data Science.
Yes, they can. With some knowledge of statistics and mathematics added to their skillset of programming languages such as Python and R, a coder can become a data scientist.
Take control of your career and land your dream job!
Sign up and start applying to the best opportunities!
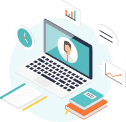